Data Types ใน python มีอะไรบ้าง
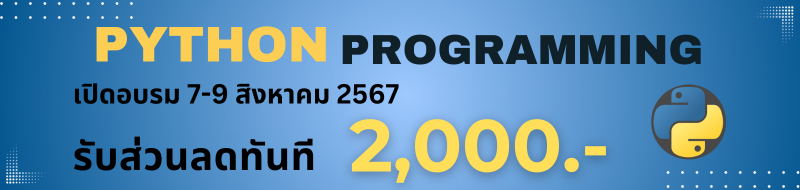
Data types คือสิ่งที่ใช้ในการกำหนดประเภท(type)ของตัวแปร ซึ่งเราสามารถตรวจสอบประเภทของตัวแปรได้ด้วย function type()
รู้จักกับ type()
type() จะใช้ตรวจสอบประเภทของตัวแปร ซึ่ง type() จะมีการรับ parameter เป็นชื่อตัวแปรที่เราต้องการตรวจสอบ เช่น
numbers = [1, 2, 3, 4]
print(type(numbers))
ผลลัพธ์จะออกมาแบบนี้
<class 'list'>
type() นอกจากจะใช้ตรวจสอบ data type แล้วยังสามารถใช้สร้าง type ขึ้นมาใหม่ก็ได้
Python data types
data types ใน python จะถูกแบ่งออกเป็นกลุ่มต่างๆ ดังนี้
- Numeric data types ประกอบไปด้วย int, float, complex
- Boolean types มี type เดียวคือ bool
- String data types มี type เดียวคือ str
- Binary types ประกอบไปด้วย bytes, bytearray, memoryview
- Sequence types ประกอบไปด้วย list, tuple
- Mapping data types มี type เดียวคือ dict
- Set data types ประกอบไปด้วย set, frozenset
- None types มี type เดียวคือ None
1. Numeric data types
เป็น data type ที่ใช้เก็บตัวเลข ซึ่งจะประกอบไปด้วย
-
int เก็บเลขจำนวนเต็ม เป็นได้ทั้ง + และ - (signed) เราสามารถประกาศตัวแปร int ได้หลายรูปแบบ ดังนี่้
a = 100 b = 0b100 # เป็นเลขฐาน 2 มีค่าเท่ากับ 4 c = 0o100 # เป็นเลขฐาน 8 มีค่าเท่ากับ 64 d = 0x100 # เป็นเลขฐาน 16 มีค่าเท่ากับ 256
นอกจากนี้เรายังสามารถ cast ตัวแปรประเภทอื่นๆ ให้กลายเป็นจำนวนเต็มได้ด้วย function int() แบบนี้
a = int(3.5) # ตัวแปร a จะมีค่าเป็น 3 b = int(-3.5) # ตัวแปร b จะมีค่าเป็น -3 c = int("496") # ตัวแปร c จะมีค่าเป็น 496 d = int("100", 8) # ตัวแปร d จะมีค่าเป็น 64 (100 ฐาน 8)
python 2 จะมี int และ long แต่ใน python 3 จะเหลือแต่ int อย่างเดียว
-
float เก็บเลขทศนิยม เราสามารถประกาศตัวแปร float ได้แบบนี้
a = 3.125 b = 3e8 # 3 x 10 ยกกำลัง 8 มีค่าเป็น 300000000.0 c = 1.616e-3 # 1.616 x 10 ยกกำลัง -3 มีค่าเป็น 0.001616
และเรายังสามารถใช้ float() ในการแปลงตัวแปรประเภทอื่นๆ มาเป็นเลขทศนิยม ได้แบบนี้
a = float("1.616") # ตัวแปร a จะมีค่าเป็น 1.616 b = float(7) # ตัวแปร b จะมีค่าเป็น 7.0 c = float("nan") # ตัวแปร c จะมีค่าเป็น Not an Number d = float("inf") # ตัวแปร d จะมีค่าเป็น Infinity e = float("-inf") # ตัวแปร e จะมีค่าเป็น -Infinity
-
complex คือ data type ที่จัดเก็บจำนวนเชิงซ้อน(complex number) แต่เราจะเปลี่ยนจาก i ไปเป็น j หรือ J แทน
a = 10 + 3j b = complex(10 + 3j) c = complex(10, 3)
ซึ่งเราจะสามารถนำไปคำนวนต่อได้แบบนี้
a = 10 + 2j b = 20 + 3j print(a + b) # ผลลัพธ์จะออกมาเป็น 30 + 5j print(a * b) # ผลลัพธ์จะออกมาเป็น 194 + 70j c = a.conjugate() # ตัวแปร c จะมีค่าเป็น 10 - 2j d = a.real # ตัวแปร d จะมีค่าเป็น 10.0 e = a.imag # ตัวแปร e จะมีค่าเป็น 2.0
2. Boolean types
เป็นตัวแปรที่เก็บผลลพธ์ทางตรรกศาสตร์ คือ จริง(True) และ เท็จ(False) ความยากของ boolean คือเราต้องรู้ว่าค่าไหนเป็น truthy หรือ falsy ซึ่ง
- truthy คือ ค่าที่เทียบเท่า True
- falsy คือ ค่าที่เทียบเท่า False
ใน python ค่าที่เทียบเท่า True(Truthy) มีค่อนข้างเยอะ ตัวอย่างของ Truthy มีดังนี้
a = bool(42) # ตัวแปร a จะมีค่าเป็น True
a = bool(-1) # ตัวแปร a จะมีค่าเป็น True
a = bool([1,2]) # ตัวแปร a จะมีค่าเป็น True
a = bool("False") # ตัวแปร a จะมีค่าเป็น True
ใน python ค่าที่เทียบเท่า False หรือ Falsy มีดังนี้
a = bool(0.0) # ตัวแปร a จะมีค่าเป็น False
a = bool(0j) # ตัวแปร a จะมีค่าเป็น False (complex number)
a = bool(None) # ตัวแปร a จะมีค่าเป็น False
a = bool([]) # ตัวแปร a จะมีค่าเป็น False (Empty list)
a = bool(()) # ตัวแปร a จะมีค่าเป็น False (Empty tuple)
a = bool({}) # ตัวแปร a จะมีค่าเป็น False (Empty Dict)
a = bool("") # ตัวแปร a จะมีค่าเป็น False (Empty string)
a = bool(range(0)) # ตัวแปร a จะมีค่าเป็น False (range(0,0) ไม่มีค่าเลย)
3. String types
ตัวแปร string คือ ตัวอักษร unicode ที่เรียงต่อกัน เราสามารถประกาศตัวแปร string ได้แบบนี้
a = 'This is a string'
a = "This is a string"
a = """This is a
multiline string"""
a = 'this is a \n multiline string'
print(a) # ข้อความ a จะขึ้นบรรทัดใหม่
เราสามารถต่อ string ได้ด้วยวิธีนี้
a = "Hello " "world" # ผลลัพธ์ออกมาเป็น 'Hello world'
a = "Hello " + "world" # ผลลัพธ์ออกมาเป็น 'Hello world'
hello = ' '.join(['Welcome','to','python','world'])
# ผลลัพธ์จะออกมาเป็น 'Welcome to python world'
colors = ';'.join(['#45ff23','#11FF23', '#CCFF44'])
# ผลลัพธ์จะออกมาเป็น '#45ff23;#11FF23;#CCFF44'
หรือเราจะ split string ออกมาแบบนี้ก็ได้
colors.split(';')
# ผลลัพธ์จะกลับมาเป็น ['#45ff23','#11FF23', '#CCFF44']
ในกรณีที่มีอักขระพิเศษ เราจะใช้ “r” นำหน้าแบบนี้ (r ย่อมาจาก raw string)
path = r"C:\Users\Training\Documents"
ผลลัพธ์จะออกมาเป็นแบบนี้
'C:\\Users\\Training\\Documents'
การจัด format ของ string เราสามารถใช้รูปแบบต่างๆ เหล่านี้
- String formatting เราจะใช้ function format ในการส่งค่าเข้าไปวางใน string
"The age of {0} is {1}".format('John', 25) # ผลลัพธ์จะออกมาเป็น 'The age of John is 25'
- F string syntax เมื่อเราใส่ “f” หน้า string เราจะสา่มารถใส่ expression ลงไปใน string นี้ได้เลย เช่น
f"one plus one is {1 + 1}" # ผลลัพธ์จะออกมาเป็น 'one plus one is 2'
4. Binary types
เป็นกลุ่มของตัวแปรที่เก็บเป็น binary ประกอบไปด้วย
- bytes เป็น data type ที่เก็บ list ของ byte(binary) โดยปกติ string ใน python จะเก็บเป็น utf-8 เมื่อแปลงเป็น bytes จะต้องเก็บอยู่ในรูปของ binary
a = b"Hello world" # ต้องมี b นำหน้า print(type(a)) # ผลลัพธ์จะออกมาเป็น <class 'bytes'> # encode กลับไปเป็น string b = a.decode('utf8') print(type(b)) # ผลลัพธ์จะออกมาเป็น <class 'str'>
- bytearray จะ return list ของ byte
numbers = [1, 2, 3, 4] a = bytearray(numbers) print(a) # ผลลัพธ์จะออกมาเป็น bytearray(b'\x01\x02\x03\x04')
- memoryview เป็นข้อมูลที่เก็บที่อยุ่(address ใน memory)
# ต่อจากตัวอย่างที่แล้ว mv = memoryview(a) # แปลง bytearray ไปเป็น memoryview # mv จะเป็น pointer ที่ชี้ไปยังจุดเริ่มต้นของจ้อมูลใน memory print(mv[0]) # mv[0] จะเป็น address ของสมาชิกตัวแรกของ bytearray # ผลลัพธ์จะออกมาเป็น 1
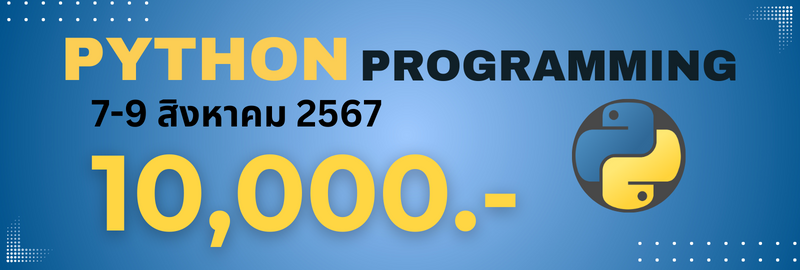
5. Sequence types
เป็นตัวแปรที่สามารถวนลูปเข้าไปในสมาชิกแต่ละตัวได้ ซึ่งประกอบไปด้วย
-
list จะเป็นเหมือนกับ array ในภาษาอื่นๆ แต่จะแตกต่างจาก array ตรงที่ list สามารถมี data type ได้มากกว่า 1 ซึ่ง list ถืิอว่าเป็น data type ที่ใช้เยอะมากๆ ใน python
# list of integers a = [1, 2, 3, 4, 5] # list of strings and integers a = ["hello", 1, 2, 3] a = list("characters") # ผลลัพธ์จะออกมาเป็น ['c', 'h', 'a', 'r', 'a', 'c', 't', 'e', 'r', 's']
อ่านการทำงานกับ python list เพิ่มเติมได้ที่นี่
-
tuple คือการเก็บข้อมูลหลายๆประเภทไว้ในตัวแปรเดียว ให้นึกถึง 1 แถวใน database ซึ่ง tuple จะเป็นตัวแปรแบบ immutabled ไม่สามารถเปลี่ยนแปลงค่าของสมาชิกแต่ละตัวได้ ถ้าจะแก้ไขต้องสร้างใหม่ทั้ง tuple
person = ("john", "doe", 25) print(person)
6. Mapping type
ใน Mapping type จะมีอยู่ประเภทเดียวคือ Dictionary ซึ่งตัวแปรประเภท Dictionary จะเหมือนกับ JSON ใน Javascript ซึ่งจะ map ระหว่าง key และ value บางครี้งอาจเรียกว่า maps หรือ associative array
contacts = {} # ประกาศ empty dict
contacts = {'bob': '081-111-2222', 'alice': '086-333-4444'}
print(contacts['bob'])
# add สมาชิกใหม่เข้าไปได้เลย
contacts['jenny'] = '062-444-5555'
# ผลลัพธ์ที่ได้จะเป็นแบบนี้
{'bob': '081-111-2222', 'alice': '086-333-4444', 'jenny': '062-444-5555'}
7. Set data types
เป็น data type ที่ใช้ที่เก็บข้อมูล โดย set จะมีข้อกำหนด ดังนี้
- สมาชิกแต่ละตัวจะไม่ซ้ำกัน
- ใน set ลำดับไม่มีความสำคัญ
เราจะใช้ set เมื่อเราต้องการใช้ operation ทางคณิตศาสตร์ เช่น union, intersection และ diff
Set data types จะแบ่งออกเป็น
- set ซึ่งจะเป็น data type ที่ mutable ซึ่งหมายถึง การเพิ่ม, ลบ หรือเปลี่ยนแปลงสมาชิกภายใน set ได้
- forzenset จะเป็น immutable หมายถึง ไม่สามารถเปลี่ยนแปลงสมาชิกได้่ ดังนั้่น forzenset จะไม่มี method add และ function อื่นๆที่เป็นการแก้ไข forzenset นี้ ดังนั้นถ้าเรา run code นี้
mylist = ['apple', 'banana', 'cherry']
x = frozenset(mylist)
x.add('pipeapple')
จะเกิด Error ขึ้นแบบนี้
ERROR!
Traceback (most recent call last):
File "<string>", line 4, in <module>
AttributeError: 'frozenset' object has no attribute 'add'
8. None types
None หรือ Nonetype จะเป็นเหมือนกับ null ในภาษาอื่นๆ คือเรายังไม่รู้ว่าข้อมูลคืออะไร เลยยังไม่สามารถระบุได้ว่าเป็น data type ประเภทไหน การกำหนดค่า None สามารถทำได้แบบนี้
a = None # ต้องเป็น N ใหญ่เท่านั้น
print(type(a))
ผลลัพธ์จะออกมาเป็นแบบนี้
<class 'NoneType'>
เวลาเปรียบเทียบค่า None ต้องระวัง เพราะ None ไม่ใช่ False แต่มีค่าเทียบเท่า False
a = None
if not a:
print('a is False')
การใข้ Union types
เราสามารถใช้ bitwise operator(|) ในการรวม data types เข้าด้วยกัน เช่น เรามีตัวแปรที่สามารถเป็นได้ทั้งจำนวนเต็ม หรือเลขทศนิยมก็ได้
ลองดูตัวอย่าง function square นี้เราสามารถส่งตัวแปร number เข้ามาเป็น int หรือ float ก็ได่้
def square(number: int | float) -> int | float:
return number ** 2
ดังนั้นใน function square นี้จะ
- return int เมื่อเราส่ง parameter เข้ามาเป็น int
- return float เมื่อเราส่ง parameter เข้ามาเป็น float
ลำดับก่อนหลังของ data type ไม่มีผล int | float มีค่าเท่ากับ float | int
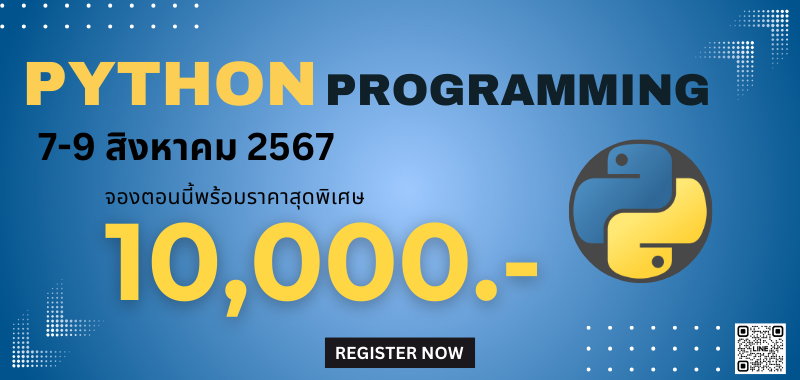